
STM32 USB_Device(CDC_Standalone)
Preface
In this article, we will explain how to use the CDC (Communication Device Class) in USB to create a virtual COM port (VCP) for communication. On the one hand, for the host computer, it appears as a serial port, and all operations are still based on serial port operations. On the other hand, the actual data transmission is based on USB, which greatly improves the data transmission speed. This article will explain the relevant content of using STM32 as a USB CDC class virtual serial port (VCP) for communication with a USB host device.
USB introduction
“In terms of USB versions, currently the STM32 series MCUs can be considered as USB 2.0 (now there is also UCPC, which has a Type-C interface for external use, but it can only be used for PD 3.0 charging and cannot be used for data communication). For STM32 series MCUs, using USB FS only requires the use of the DM/D- and DP/D+ pins, with the addition of at most three pins for ID, SOF, and VBUS. However, using USB HS mostly requires an external PHY chip (such as USB3300), which requires more pins, at least 12. When using USB functionality, it is recommended to use an external clock, such as a passive crystal oscillator or an active crystal oscillator, as USB requires a high level of clock accuracy. The STM32 CDC VCP is plug-and-play for Windows 10 and newer versions of Linux, but for older versions of Windows, drivers need to be installed. The driver download address is as follows:
STSW-STM32102 STM32 Virtual COM Port Driver
STM32CubeMX Setting
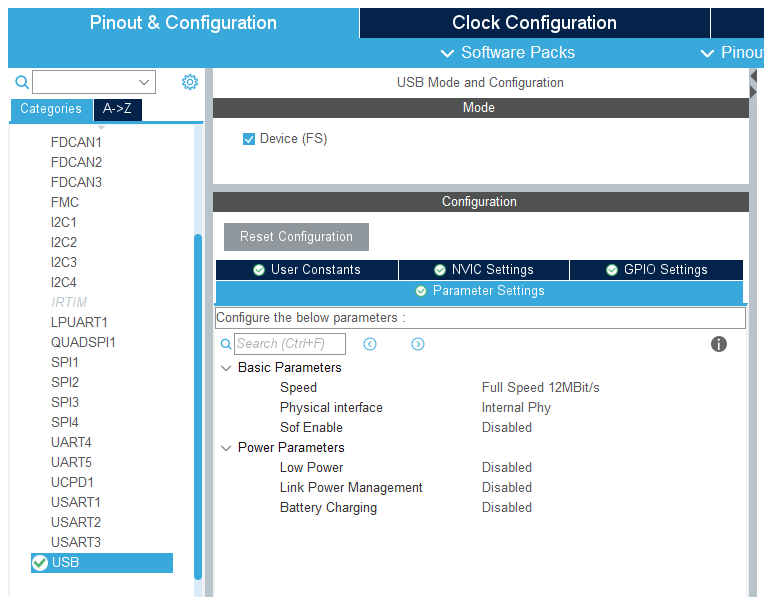
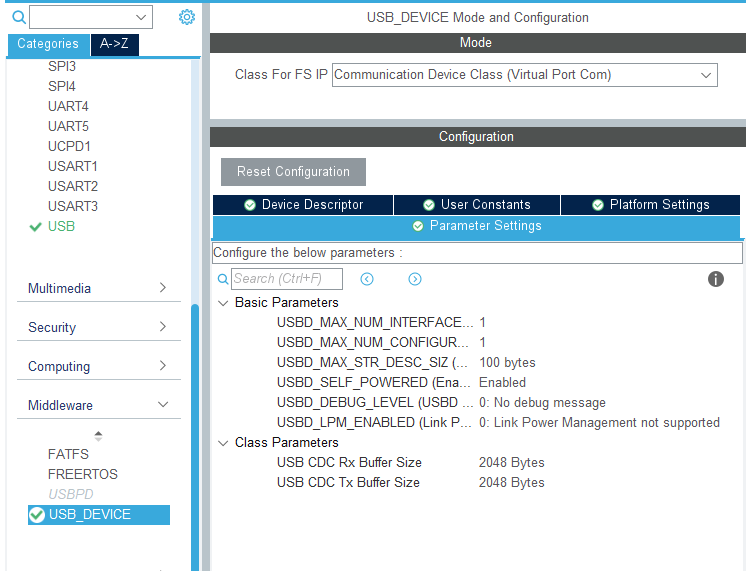
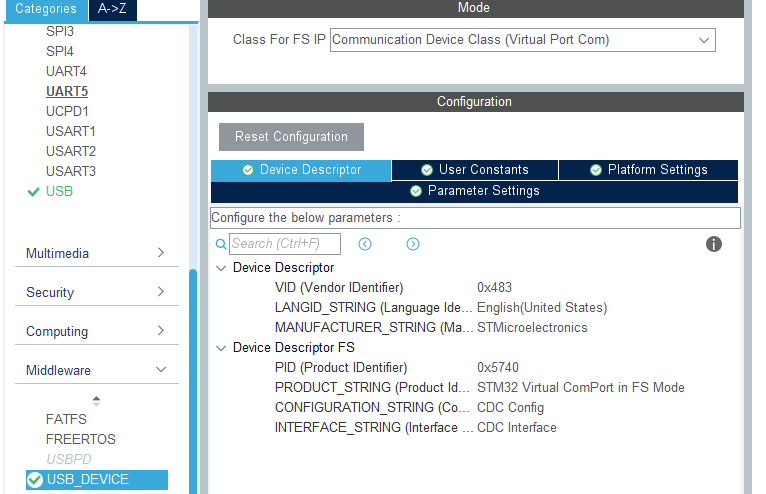
#Note that the VCP USB interrupt must be enabled in order to be recognized by the computer.

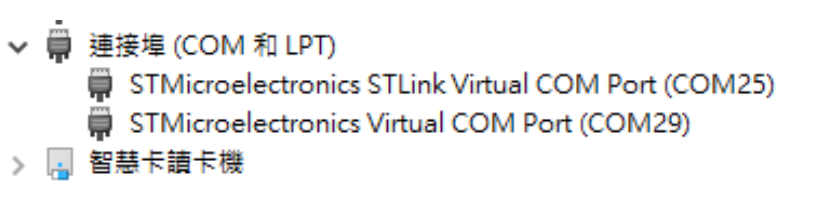
Code Structure
In the generated code for the above configuration, the USB-related code for the user is located in USB_DEVICE > App, with the most important file being usbd_cdc_if.c. In most cases, modifying this file is sufficient to meet the relevant requirements. The main structure and explanation of this file are as follows:
static uint8_t USBD_CDC_DataOut(USBD_HandleTypeDef *pdev, uint8_t epnum)
{
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *) pdev->pClassData;
/* Get the received data length */
hcdc->RxLength = USBD_LL_GetRxDataSize(pdev, epnum);
/* USB data will be immediately processed, this allow next USB traffic being
NAKed till the end of the application Xfer */
if (pdev->pClassData != NULL)
{
((USBD_CDC_ItfTypeDef *)pdev->pUserData)->Receive(hcdc->RxBuffer, &hcdc->RxLength);
return USBD_OK;
}
else
{
return USBD_FAIL;
}
}
- CDC_Control_FS() Callback functions from the host
- CDC_Receive_FS() Callback function for receiving data;
- CDC_Transmit_FS() Callback function for sending data;
- CDC_TransmitCplt_FS() Callback function for sending completion;