
AMS TMF8801 (arduino/STM32)-1
Preface
A time-of-flight camera (ToF camera) is a range imaging camera system employing time-of-flight techniques to resolve distance between the camera and the subject for each point of the image, by measuring the round trip time of an artificial light signal provided by a laser or an LED.
We chose the SparkFun Qwiic Time-of-Flight Sensor TMF8801 module as the driving template, paired with the STM32F401RE Nucleo-F401RE board.


We are using the TMF8801 and Nucleo-F401RE as development tools. The following are programming examples and wiring instructions.
TMF8801 Introduce
The TMF8801 is an integrated module launched by AMS in 2019 for distance measurement (dToF), claiming to be the smallest module at the time. This sensor can accurately measure distances ranging from 0.02m to 2.5m. In actual measurement, it can measure up to a maximum of 1m with additional devices, and in a dark room, it can measure up to 2.5m. Compared to the VL53L0X distance measuring module, the measurement range and accuracy of the TMF8801 are relatively better, and it can measure distances within 2m with good accuracy.

Features
- Direct ToF technology with high sensitivity SPAD detection
- Fast Time-to-Digital Converter (TDC) architecture
- Sub-nanosecond light pulse
- 20 – 2500mm distance sensing @ 30Hz
- On-chip histogram processing
- 940nm VCSEL Class 1 Eye Safety
- High performance on-chip sunlight rejection filter and algorithm
- Industry’s smallest modular OLGA 2.2 x 3.6 x 1.0 mm package
Benefits
- Delivers high SNR, wide dynamic range and no multi-path reflections
- Enables dark and sunlight environment distance measurement within ±5%
- Provides best-in-class resolution ranging mode detection sensing
- Enables accurate distance measurements
- Provides high accuracy, greater distance between cover glass, dynamic cover glass calibration, dirt or smudge removal and crosstalk compensation
- Eye-safety circuitry stops VCSEL driver if VCSEL fault occurs
- Optical filters with algorithm support enables high ambient light resilience
- Reduce board space requirements, enables low-profile system designs in restricted space Industrial Designs
Connection layout

The hardware part is completed by connecting the sensor board to the corresponding pins of the Nucleo-F401RE board shown in the figure above.
Note that the sensor board operates at 3.3V, not 5V, and its I2C pins already contain resistors. If you are soldering your own circuit board, be sure to add external resistors to ensure the signal is normal.
Coding Flow
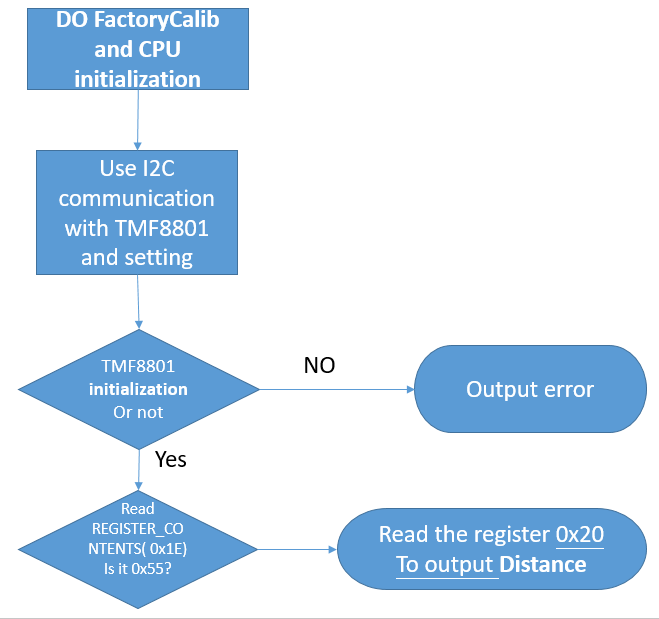
Since there are many online resources available, we won’t post all the code for interpreting the STM32 here. Instead, we will provide a few excerpts for your reference.
- Before starting sensing, the memory inside the TMF8801 must be reset to avoid causing errors in the I2C write command.
The Sensor Initialization
void reset_SW()
{
uint8_t status=0,x=0,cmd_buf[3]={0x10,0x00,0xEF},ready=-1;
write_register(APPREQID,Bootloader);
TMF8801_reset();
if (!waitForCpuReady())
{
x=read_register(ENABLE);
printf("CPU staute= %#x nr",x);
return ;}
while(1)
{
status=currentApplication();
if(status==1)//Bootloader
{
HAL_I2C_Mem_Write(&hi2c1, Devadress, 0x08, I2C_MEMADD_SIZE_8BIT, &cmd_buf,3, 100);
HAL_Delay(10);
//remap_rst_RAM();
ready=__tmf8801_status_read ();
if(ready==TMF8x01_OK)
{printf("reset SW okrn");
return;}
else if(ready==TMF8x01_NK)
{printf("reset SW failrn");
for(;;);}
}
}
}
To avoid errors in the I2C write command, the memory inside the TMF8801 must be reset before starting sensing. Basically, the process involves switching to the bootloader state to restart the TMF8801’s firmware, so that all states are in their original state.
FactoryCalib
void TMF8801_FactoryCalib()
{
uint8_t y=0,z=0;
printf("wait TMF8801 Factory Calibrationnr");
if (read_register(ID)!=0x07) {
y=read_register(ID);
printf("chipy ID= %#x nr",y);
printf("chip ID errornr");
return;
};
TMF8801_loadApplication();
if (!waitForApplication())
{
z=read_register(APPID);
printf("Application staute= %#x errornr",z);
return;
}
write_register( COMMAND,FACTORY_CALIB);
while(1){
// write_register( COMMAND,FACTORY_CALIB);
if(read_register(0x1E)==0x0A)
{ printf("Calibration completenr");
HAL_I2C_Mem_Read(&hi2c1,Devadress,0x20, I2C_MEMADD_SIZE_8BIT, &factory_calib_data, 14, 100);
//FACTORY_CALIB_0 = 0x20
return ;
}
HAL_Delay(20);
}
};
This step (FactoryCalib) can be skipped, but you need to input an array beforehand to ensure that the initialization can proceed smoothly
Sensor Initialization settings.
write_register(COMMAND,DOWNLOAD_CALIB_AND_STATE);//COMMAND = 0x10/DOWNLOAD_CALIB_AND_STATE = 0x0B
HAL_I2C_Mem_Write(&hi2c1,Devadress,FACTORY_CALIB_0,I2C_MEMADD_SIZE_8BIT,&factory_calib_data,14,100);//FACTORY_CALIB_0= 0x20/
HAL_I2C_Mem_Write(&hi2c1,Devadress,STATE_DATA_WR_0,I2C_MEMADD_SIZE_8BIT,TMF8801_ALGO_STATE,11,100);//STATE_DATA_WR_0= 0x2E
write_register(CMD_DATA7,0x03);//CMD_DATA7 0x08 Algorithm state and factory calibration is provided
write_register(CMD_DATA6,0xA3);//CMD 6 0xA7 long mode 0xA3 defaut
write_register(CMD_DATA5,0x00);//CMD 5 No GPIO control used
write_register(CMD_DATA4,0x00);//CMD 4 No GPIO control used
write_register(CMD_DATA3,0x00);//CMD 3 defaut 0x00 long 0x40
write_register(CMD_DATA2,measurement_period_ms);
write_register(CMD_DATA1,0xD8);//CMD 1 0x03 defaut 0x04 short mode
write_register(CMD_DATA0,0x04);//CMD 0 0x04 to actually perform the measurement
write_register(COMMAND,DISTANCE_MEASURE_MODE);//COMMAND = 0x10 /DISTANCE_MEASURE_MODE_1= 0x02
The array ‘factory_calib_data’ stores the calibration values obtained during the factory calibration. If these values are abnormal or missing, the TMF8801 will not be able to measure distance accurately
Pingback: AMS Time-of-Flight sensor ( TMF8801 )on STM32 -2
Pingback: AMS Time-of-Flight sensor ( TMF8801 )on STM32 -2